Animate.cssの使い方
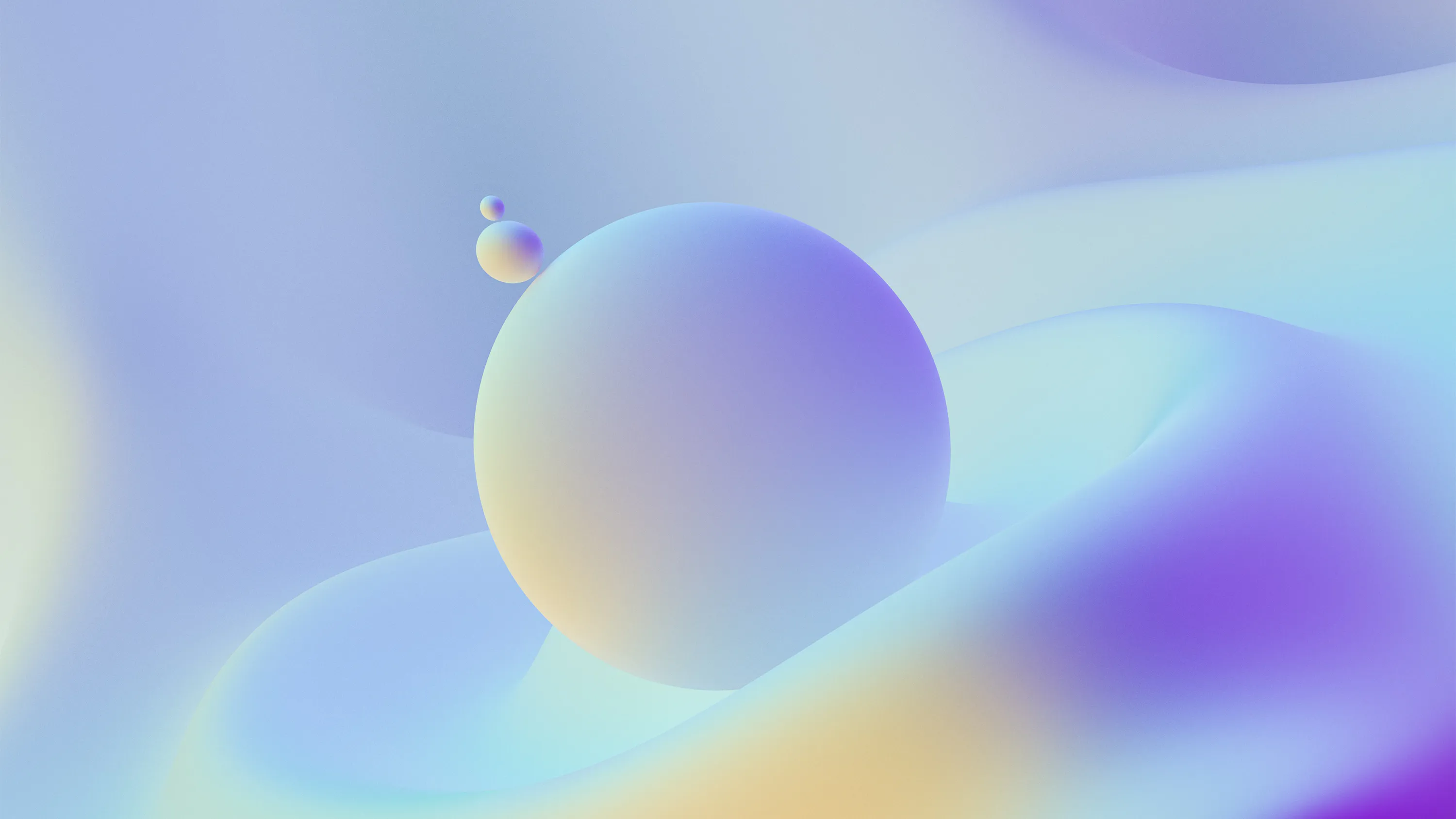
Animate.cssというcssのライブラリを紹介します。
使い方
まずはどんなアニメーションがあるのかを見てみましょう。
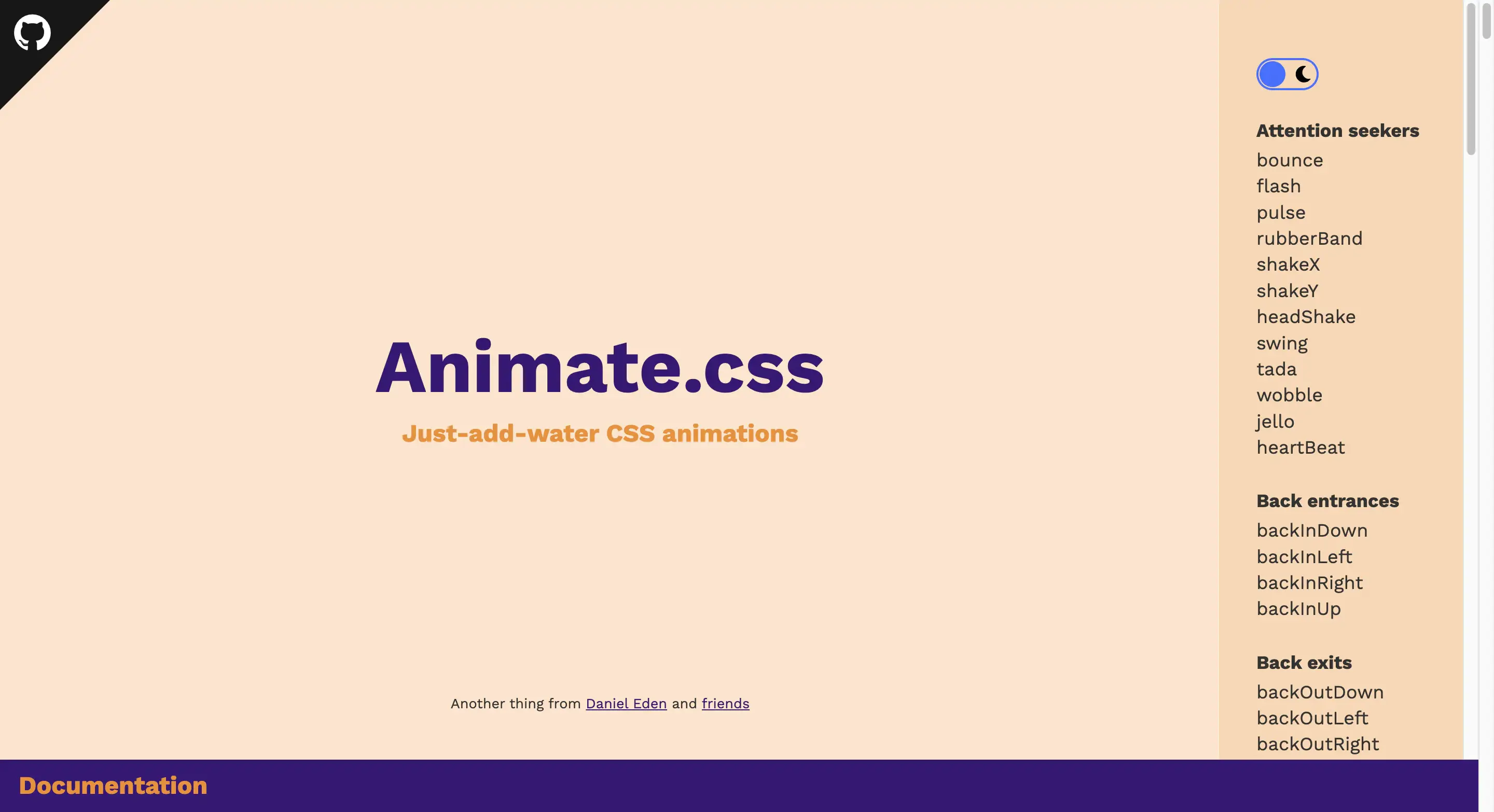
全部で97個もアニメーションがあります。
右のアニメーションの名前をクリックすると、真ん中の「Animate.css」という文字がそのアニメーションになります。
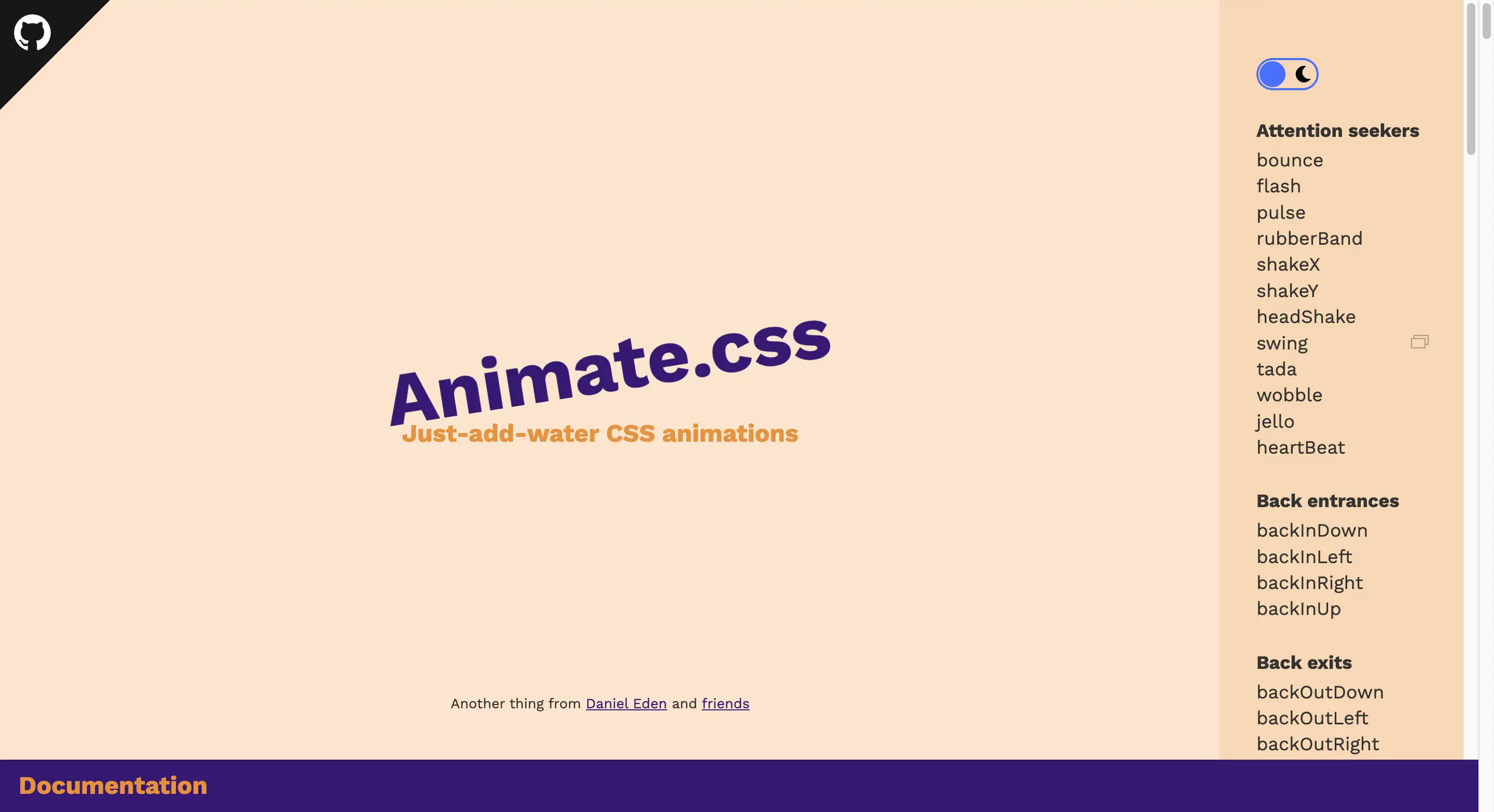
ということで実際にアニメーションを使ってみましょう。
今回は次の要素にアニメーションをつけます。
<p>animation</p>
まず、head要素内に次のコードを貼り付けてAnimate.cssを読み込んでください。
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/animate.css/4.1.1/animate.min.css"/>
次にアニメーションをつけたい要素に、
animate__animated
とつけたいアニメーションをコピーしたクラス名を付与してください。
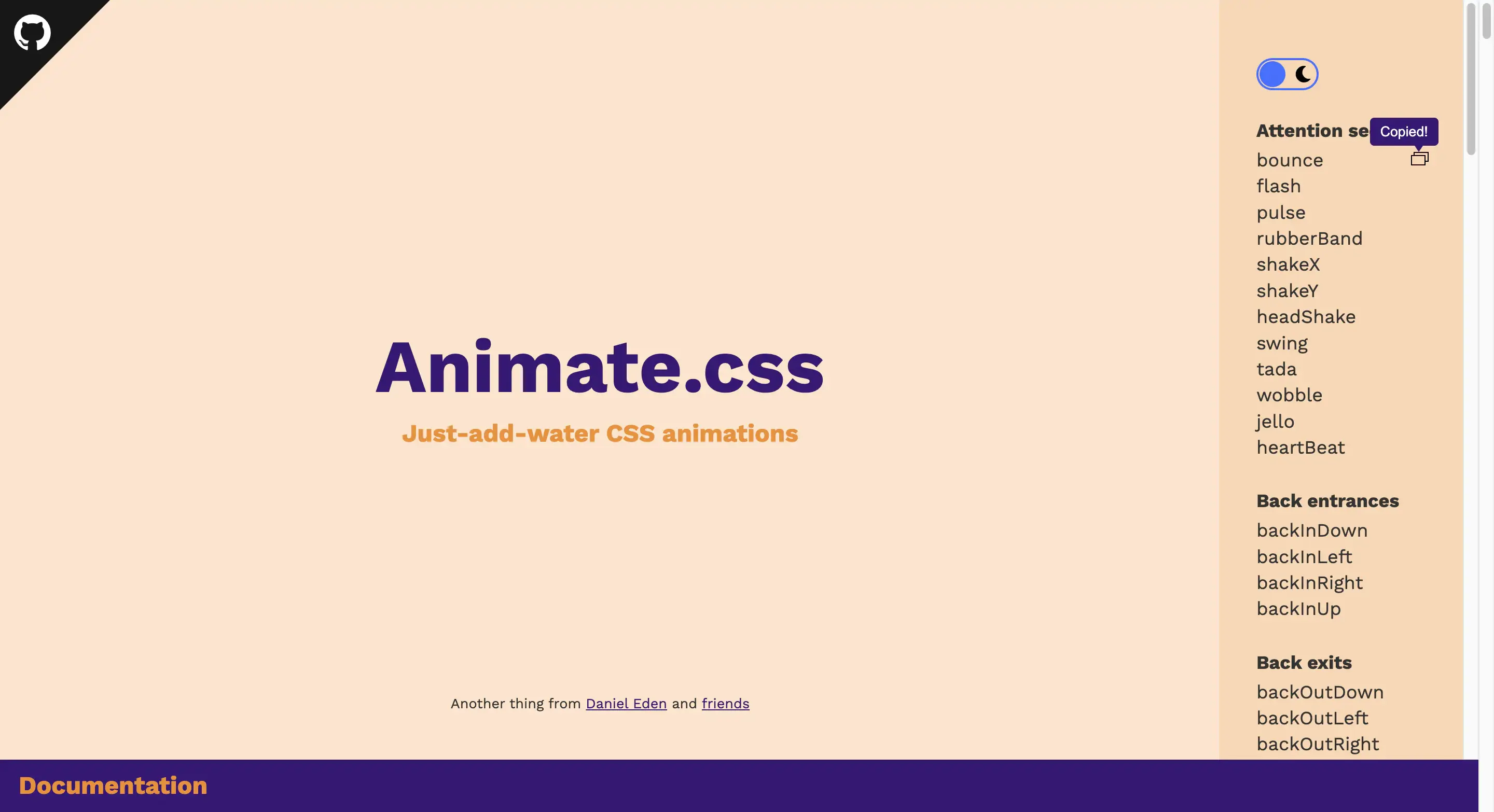
こんな感じでコピーできます。
<p class="animate__animated animate__bounce">animation</p>
これでアニメーションができました。
ここからはアニメーションの回数、速さ、遅延、画面領域に入ったら実行する方法などを紹介していきます。
回数
クラスを付与
アニメーションの回数は1 ~ 3回までは次のクラス名を付与すればOKです。
animate__repeat-1
animate__repeat-2
animate__repeat-3
css
4回以上にしたい場合はcssで次のように指定してください。
p{
animation-iteration-count: 4;
}
無限に
次のクラス名を付与すると無限にアニメーションさせることができます。
animate__infinite
速さ
アニメーションの速さを変更します。
こちらもクラス名を付与する方法と、cssで変更する方法があります。
クラスを付与
クラス名 | 速さ |
---|---|
animate__slow | 2s |
animate__slower | 3s |
animate__fast | 800ms |
animate__faster | 500ms |
css
cssで次のように指定すればOKです。
animation-duration: 2.5s;
遅延
アニメーションのスタートを遅らせます。
クラスを付与
1 ~ 5秒までは次のクラス名を付与すればできます。
animate__delay-1s
animate__delay-2s
animate__delay-3s
animate__delay-4s
animate__delay-5s
css
次のように指定すれば自由に遅延時間を決めることができます。
animation-delay: 500ms;
画面領域に入ったら
要素が画面に入ったらアニメーションを実行するようにしましょう。
次のコードでできます。
function handleIntersection(entries, observer) {
entries.forEach(entry => {
if (entry.isIntersecting) {
entry.target.classList.add('animate__bounce');
} else {
entry.target.classList.remove('animate__bounce');
}
});
}
const observer = new IntersectionObserver(handleIntersection, {
threshold: 0
});
const observedElement = document.querySelector('.animate__bounce');
observer.observe(observedElement);
上のコードでいうと、4, 6, 15行目にある「animate__bounce」は使うアニメーションによって変えてください。
また、これは要素が画面領域に入るたびに何回でもアニメーションさせるコードです。
合計で一回だけアニメーションを実行したい場合は以下のコードに変えてください。
function handleIntersection(entries, observer) {
entries.forEach(entry => {
if (entry.isIntersecting) {
entry.target.classList.add('animate__bounce');
}
});
}
const observer = new IntersectionObserver(handleIntersection, {
threshold: 0
});
const observedElements = [...document.querySelectorAll('.animate__bounce')];
observedElements.forEach( (element) => {
observer.observe(element);
element.classList.remove('animate__bounce');
})
cssのコード
今回使ったAnimate.cssのcssのソースコードはGitHubで公開されているのでみてみるのも面白いかもしれません。
ちなみにanimate__bounceのcssのソースコードはこれです。
@keyframes bounce {
from,
20%,
53%,
to {
animation-timing-function: cubic-bezier(0.215, 0.61, 0.355, 1);
transform: translate3d(0, 0, 0);
}
40%,
43% {
animation-timing-function: cubic-bezier(0.755, 0.05, 0.855, 0.06);
transform: translate3d(0, -30px, 0) scaleY(1.1);
}
70% {
animation-timing-function: cubic-bezier(0.755, 0.05, 0.855, 0.06);
transform: translate3d(0, -15px, 0) scaleY(1.05);
}
80% {
transition-timing-function: cubic-bezier(0.215, 0.61, 0.355, 1);
transform: translate3d(0, 0, 0) scaleY(0.95);
}
90% {
transform: translate3d(0, -4px, 0) scaleY(1.02);
}
}
.bounce {
animation-name: bounce;
transform-origin: center bottom;
}